package com.nio;
import org.junit.Test;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.DatagramChannel;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.util.Date;
import java.util.Iterator;
import java.util.Scanner;
public class TestUDP {
@Test
public void send() throws IOException {
//1. 得到通道
DatagramChannel dc = DatagramChannel.open();
//2. 切换到非阻塞模式
dc.configureBlocking(false);
//3. 获取缓冲区
ByteBuffer buf = ByteBuffer.allocate(1024);
Scanner scan = new Scanner(System.in);
while (scan.hasNext()){
String str = scan.next();
buf.put((new Date().toString() + ":\n" + str).getBytes());
buf.flip();
dc.send(buf,new InetSocketAddress("127.0.0.1",9898));
buf.clear();
}
dc.close();
}
@Test
public void receive() throws IOException {
DatagramChannel dc = DatagramChannel.open();
dc.configureBlocking(false);
// 绑定端口
dc.bind(new InetSocketAddress(9898));
Selector selector = Selector.open();
dc.register(selector, SelectionKey.OP_READ);
while (selector.select() > 0){
Iterator<SelectionKey> it = selector.selectedKeys().iterator();
while (it.hasNext()){
SelectionKey sk = it.next();
if (sk.isReadable()){
ByteBuffer buf = ByteBuffer.allocate(1024);
dc.receive(buf);
buf.flip();
System.out.println(new String(buf.array(),0,buf.limit()));
buf.clear();
}
}
it.remove();
}
}
}
NIOUDP方式
原创
©著作权归作者所有:来自51CTO博客作者wx5ba7ab4695f27的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:NIO的pipe管道
下一篇:MYSQLsql99语法内连接
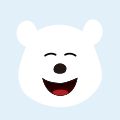
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
虚电路方式,数据报方式虚电路 数据 通信子网 连接建立 结点
-
Sentinel docker 启动 docker启动ssh
说明:我使用的是Centos安装docker第一步:安装dockersudo yum install -y yum-utilssudo yum-config-manager --add-repo https://download.daocloud.io/docker/linux/centos/docker-ce.reposudo yum install -y -q --setopt
Sentinel docker 启动 安装docker ssh登录docker容器 Linux系统 docker