package com.zyd.billondataCollect;
import com.alibaba.fastjson.JSONObject;
import com.zyd.input.app.AppProductLog;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Calendar;
import java.util.Date;
import java.util.Random;
/**
* 模拟http请求类
*/
public class AppGenerateData {
private static Random random = new Random();
private static int[] days = new int[]{2, 4, 3, 5};
private static String[] userIds = new String[]{"1", "2"}; //用户id
private static String[] deviceIds = new String[]{"deviceId1", "deviceId2"}; //设备id
private static String openTimeStamp = generateTime(new Date()) + ""; //打开时间
private static String leaveTimeStamp = (generateTime(new Date()) + 10 * 1000) + ""; //退出时间
private static String[] appPlatforms = new String[]{"andriod", "ios"}; //平台 : 安卓/IOS
private static String[] deviceStyle = new String[]{"iphone11", "小米10", "iphone5s", "荣耀10"}; //型号
private static String[] brands = new String[]{"华为", "小米", "苹果"}; //品牌
private static String[] screenSizes = new String[]{"200*300", "300*500", "400*200"}; //分辨率
private static String[] osTypes = new String[]{"andriod4.5", "andriod5.6", "ios5", "ios6", "ios7"}; //操作系统
private static String[] channelIds = new String[]{"1", "2"}; //频道id
private static String[] productTypeId = new String[]{"2", "3"}; //产品类别id
private static String[] productId = new String[]{"1", "2", "3"}; //产品id
private static String scanTime = generateTime(new Date()) + ""; //浏览时间
private static String jumpTime = (generateTime(new Date()) + 5 * 1000) + ""; //跳出商品时间
private static String[] ips = new String[]{"192.168.1.2", "192.168.1.3", "192.168.18.5"}; //用户app访问的ip
private static String[] country = new String[]{"china"}; //国家
private static String[] provinces = new String[]{"安徽", "上海", "广东", "湖南"}; //省
private static String[] cities = new String[]{"芜湖市", "南京市", "无锡市", "深圳市"}; //市
private static String[] networks = new String[]{"Wifi", "4G", "5G"}; //网络方式
private static String[] operators = new String[]{"移动", "联通", "电信"}; //运营商
private static String[] appVersions = new String[]{"2.3.1", "2.4.1", "3.0.2"}; //app版本
private static String[] appChannels = new String[]{"安卓市场", "小米市场", "华为市场"}; //渠道
private static AppProductLog generateData() {
AppProductLog appProductLog = new AppProductLog();
appProductLog.setUserId(userIds[random.nextInt(userIds.length)]);
appProductLog.setDeviceId(deviceIds[random.nextInt(deviceIds.length)]);
appProductLog.setAppPlatform(appPlatforms[random.nextInt(appPlatforms.length)]);
appProductLog.setDeviceStyle(deviceStyle[random.nextInt(deviceStyle.length)]);
appProductLog.setBrand(brands[random.nextInt(brands.length)]);
appProductLog.setScreenSize(screenSizes[random.nextInt(screenSizes.length)]);
appProductLog.setOsType(osTypes[random.nextInt(osTypes.length)]);
appProductLog.setProductId(productId[random.nextInt(productId.length)]);
appProductLog.setProductTypeId(productTypeId[random.nextInt(productTypeId.length)]);
appProductLog.setChannelId(channelIds[random.nextInt(channelIds.length)]);
appProductLog.setIp(ips[random.nextInt(ips.length)]);
appProductLog.setCountry(country[random.nextInt(country.length)]);
appProductLog.setProvince(provinces[random.nextInt(provinces.length)]);
appProductLog.setCity(cities[random.nextInt(cities.length)]);
appProductLog.setNetwork(networks[random.nextInt(networks.length)]);
appProductLog.setOperator(operators[random.nextInt(operators.length)]);
appProductLog.setAppVersion(appVersions[random.nextInt(appVersions.length)]);
appProductLog.setAppChannel(appChannels[random.nextInt(appChannels.length)]);
appProductLog.setOpenTimeStamp(openTimeStamp);
appProductLog.setLeaveTimeStamp(leaveTimeStamp);
appProductLog.setScanTime(scanTime);
appProductLog.setJumpTime(jumpTime);
return appProductLog;
}
private static long generateTime(Date date) {
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(Calendar.DAY_OF_MONTH, -days[random.nextInt(days.length)]);
long resultTime = calendar.getTime().getTime();
return resultTime;
}
private static void postHttpMethod(String urlPath, String data) {
try {
URL url = new URL(urlPath);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
//请求方式
urlConnection.setRequestMethod("POST");
//支持输入输出
urlConnection.setDoInput(true);
urlConnection.setDoOutput(true);
//是否支持重定向
urlConnection.setInstanceFollowRedirects(true);
//使用缓存
urlConnection.setUseCaches(true);
//设置请求头信息
// urlConnection.setRequestProperty("User-Agent","Mozilla/5.0(Windows NT 6.1;Win64; x64; rv:50.0) Gecko/20100101 Firefox/50.0");
urlConnection.setRequestProperty("User-Agent", "Mozilla/5.0(Windows NT 6.1;Win64; x64; rv:50.0) Gecko/20100101 Firefox/50.0");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.setConnectTimeout(5 * 1000);
//连接
urlConnection.connect();
//设置输出流,向文件中写入
OutputStream outputStream = urlConnection.getOutputStream();
outputStream.write(data.getBytes());
//刷新缓冲区
outputStream.flush();
outputStream.close();
//输入流
InputStream inputStream = urlConnection.getInputStream();
//请求返回状态码
int httpCode = urlConnection.getResponseCode();
byte[] inputData = new byte[1024];
StringBuffer stringBuffer = new StringBuffer();
while (inputStream.read(inputData, 0, 1024) != -1) {
stringBuffer.append(new String(inputData));
}
System.out.println("状态码:" + httpCode);
System.out.println("消息:" + stringBuffer.toString());
inputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
AppProductLog appProductLog = generateData();
System.out.println(JSONObject.toJSONString(appProductLog));
postHttpMethod("http://localhost:8091/collectAppData", JSONObject.toJSONString(appProductLog).toString());
}
}
高性能实时数仓建设(四):模拟数据代码
原创wx5ba7ab4695f27 ©著作权
文章标签 Kylin 数仓建设 数据代码 文章分类 大数据
上一篇:Ranger(一)初步感知
下一篇:开发者学习交流
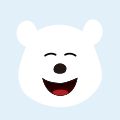
-
实时数仓建设
实时数仓建设
数据 离线 数据源 -
大数据之实时数仓建设(一)
一、数据采集服务
数据仓库 kafka flink apache -
详解大厂实时数仓建设方案
一、实时数仓建设背景1. 实时需求日趋迫切目前各大公司的产品需求和内部决策对于数据实时性的要求越来越迫切,需要实
大数据 数据库 数据 离线 数据源 -
伴鱼实时数仓建设案例
随着伴鱼业务的快速发展,离线数据日渐无法满足运营同学的需求,数据的实时性要求越来越高。之前的实时任务是通过实时
数据仓库 数据 Redis 缓存 -
mysql select count 默认值
问题描述 需求:查询出每月 order_amount(订单金额) 排行前3的记录。例如对于2019-02,查询结果中就应该是这3条: 解决方法MySQL 5.7 和 MySQL 8.0 有不同的处理方法。1. MySQL 5.7我们先写一个查询语句。根据 order_date 中的年、月,和order_amount进行降序排列。然后,添加一个新列:order_amount(
mysql 查询不到记录取默认值 mysql 每个分类取5条 mysql分组查询 mysql查询本月第一周 order by 子查询