using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Runtime.InteropServices; //调用动态库一定要加入这个引用
using System.Text;//一定要加入这个
namespace PayTest
{
public partial class Form1 : Form
{
[DllImport("PayApiFun.dll", EntryPoint = "StrAddStr", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int StrAddStr(string inputstr,ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "PQRCodeEx", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int PQRCodeEx(string inputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinPayCodeEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinPayCodeEx1(string Lockappid, string Lockmchid, string Idkey, int IdkeyLock, string AuthCode, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinPayQRCodeEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinPayQRCodeEx1(string Lockappid, string Lockmchid, string Idkey, int IdkeyLock, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinQueryEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinQueryEx1(string Lockappid, string Lockmchid, string Idkey, int IdkeyLock, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinCloseOrderEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinCloseOrderEx1(string Lockappid, string Lockmchid, string Idkey, int IdkeyLock, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoPayCodeEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoPayCodeEx1(string Lockappid, string AuthCode, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoPayQRCodeEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoPayQRCodeEx1(string Lockappid, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoQueryEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoQueryEx1(string Lockappid, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoPayCancelEx1", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoPayCancelEx1(string Lockappid, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinPayCodeEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinPayCodeEx2(string appid, string mchid, int registered, string Idkey, int IdkeyLock, string AuthCode, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinPayQRCodeEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinPayQRCodeEx2(string appid, string mchid, int registered, string Idkey, int IdkeyLock, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinQueryEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinQueryEx2(string appid, string mchid, int registered, string Idkey, int IdkeyLock, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "WeiXinCloseOrderEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int WeiXinCloseOrderEx2(string appid, string mchid, int registered, string Idkey, int IdkeyLock, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoPayCodeEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoPayCodeEx2(string appid, int registered, string AuthCode, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoPayQRCodeEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoPayQRCodeEx2(string appid, int registered, string OrdNum, string TotalFee, string inbody, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoQueryEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoQueryEx2(string appid, int registered, string OrdNum, ref IntPtr outputstr);
[DllImport("PayApiFun.dll", EntryPoint = "ZhiFuBaoPayCancelEx2", CharSet = CharSet.Ansi, CallingConvention = CallingConvention.StdCall)]
static extern int ZhiFuBaoPayCancelEx2(string appid, int registered, string OrdNum, ref IntPtr outputstr);
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
}
private void button2_Click(object sender, EventArgs e)
{
string lockappid = WXAPPID.Text.Trim(); //微信公众号
string lockmachid = WXMCHID.Text.Trim(); //商户号
int registered = 0; //registered = 0 原始的公众号、商户号 =1 已加密授权的公众号、商户号
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string idkey = WXKEY.Text.Trim(); //API密钥
int lockkey = 0; //lockkey = 0 原始API密钥 =1 加密后的API密钥
if (radioButton1.Checked == true) { lockkey = 0; } else { lockkey = 1; }
string OrdNum = textBox2.Text.Trim();
string TotalFee = textBox3.Text.Trim();
string inbody = textBox6.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
textBox1.Text = "";
textBox5.Text = "";
button2.Text = "正在申请支付URL...";
Application.DoEvents();
//int real = WeiXinPayQRCodeEx1(lockappid, lockmachid, idkey, lockkey, OrdNum,TotalFee,inbody, ref outputs); //第一代函数
int real = WeiXinPayQRCodeEx2(lockappid, lockmachid,registered, idkey, lockkey, OrdNum,TotalFee,inbody, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "微信支付单号:" + OrdNum + ",支付二维码已经生成,有效时间5分钟,可轮询调用‘查询微信订单状态’函数查询支付结果!";
textBox5.Text = Marshal.PtrToStringAnsi(outputs).ToString();
pictureBox2.ImageLocation = Environment.CurrentDirectory + "\\QRCode.bmp";
break;
case 1:
textBox1.Text = "微信支付单号:" + OrdNum + ",正在申请支付二维码,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
case -10:
textBox1.Text = "微信支付单号:" + OrdNum + ",支付二维码URL:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default :
textBox1.Text = "微信支付单号:" + OrdNum + ",申请支付URL失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button2.Text = "生成微信支付二维码 Ex1";
}
private void Form1_Load(object sender, EventArgs e)
{
pictureBox2.ImageLocation = Environment.CurrentDirectory + "\\QRCode.bmp";
textBox2.Text = DateTime.Now.ToString("yyyyMMddHHMMss")+"0001";
}
private void button4_Click(object sender, EventArgs e)
{
string lockappid = WXAPPID.Text.Trim(); //微信公众号
string lockmachid = WXMCHID.Text.Trim(); //商户号
int registered = 0; //registered = 0 原始的公众号、商户号 =1 已加密授权的公众号、商户号
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string idkey = WXKEY.Text.Trim(); //API密钥
int lockkey = 0; //lockkey = 0 原始API密钥 =1 加密后的API密钥
if (radioButton1.Checked == true) { lockkey = 0; } else { lockkey = 1; }
string OrdNum = textBox2.Text.Trim ();
IntPtr outputs = Marshal.AllocHGlobal(255);
textBox1.Text = "";
button4.Text = "正在查询支付结果...";
Application.DoEvents();
//int real = WeiXinQueryEx1(lockappid, lockmachid, idkey, lockkey, OrdNum, ref outputs); //第一代函数
int real = WeiXinQueryEx2(lockappid, lockmachid,registered, idkey, lockkey, OrdNum, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "微信支付单号:" + OrdNum + ",支付成功,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
case 1:
textBox1.Text = "微信支付单号:" + OrdNum + ",正在支付中,请稍后查询支付状态,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default:
textBox1.Text = "微信支付单号:" + OrdNum + ",支付失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button4.Text = "查询微信订单状态 Ex1";
}
private void button9_Click(object sender, EventArgs e)
{
panel1.Visible = false;
}
private void button8_Click(object sender, EventArgs e)
{
panel1.Visible =true ;
}
private void button3_Click(object sender, EventArgs e)
{
string lockappid = WXAPPID.Text.Trim(); //微信公众号
string lockmachid = WXMCHID.Text.Trim(); //商户号
int registered = 0; //registered = 0 原始的公众号、商户号 =1 已加密授权的公众号、商户号
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string idkey = WXKEY.Text.Trim(); //API密钥
int lockkey = 0; //lockkey = 0 原始API密钥 =1 加密后的API密钥
if (radioButton1.Checked == true) { lockkey = 0; } else { lockkey = 1; }
string AuthCode = textBox4.Text.Trim();
string OrdNum = textBox2.Text.Trim();
string TotalFee = textBox3.Text.Trim();
string inbody = textBox6.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
if(AuthCode=="")
{
MessageBox.Show("请输入正确的微信付款码!", "警告", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
textBox1.Text = "";
textBox5.Text = "";
button3.Text = "正在申请微信支付...";
Application.DoEvents();
//int real = WeiXinPayCodeEx1(lockappid, lockmachid, idkey, lockkey, AuthCode, OrdNum, TotalFee, inbody, ref outputs); //第一代函数
int real = WeiXinPayCodeEx2(lockappid, lockmachid,registered, idkey, lockkey, AuthCode, OrdNum, TotalFee, inbody, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "微信支付单号:" + OrdNum + ",支付成功,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
case 1:
textBox1.Text = "微信支付单号:" + OrdNum + ",正在支付中,请稍后查询支付状态,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default:
textBox1.Text = "微信支付单号:" + OrdNum + ",支付失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button3.Text = "微信收付款码支付 Ex1";
}
private void button7_Click(object sender, EventArgs e)
{
string lockappid = ZFBAPPID.Text.Trim(); //支付宝APPID
int registered = 0; //registered = 0 原始的支付宝APPID =1 已加密授权的支付宝APPID
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string OrdNum = textBox2.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
textBox1.Text = "";
button7.Text = "正在查询支付结果...";
Application.DoEvents();
//int real = ZhiFuBaoQueryEx1(lockappid, OrdNum, ref outputs); //第一代函数
int real = ZhiFuBaoQueryEx2(lockappid,registered, OrdNum, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",支付成功,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
case 1:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",正在支付中,请稍后查询支付状态,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",支付失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button7.Text = "查询支付宝订单状态 Ex";
}
private void button6_Click(object sender, EventArgs e)
{
string lockappid = ZFBAPPID.Text.Trim(); //支付宝APPID
int registered = 0; //registered = 0 原始的支付宝APPID =1 已加密授权的支付宝APPID
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string AuthCode = textBox4.Text.Trim();
string OrdNum = textBox2.Text.Trim();
string TotalFee = (Convert.ToDouble(textBox3.Text) / 100).ToString();
string inbody = textBox6.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
if (AuthCode == "")
{
MessageBox.Show("请输入正确的支付宝付款码!", "警告", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
textBox1.Text = "";
textBox5.Text = "";
button6.Text = "正在申请支付...";
Application.DoEvents();
//int real = ZhiFuBaoPayCodeEx1(lockappid, AuthCode, OrdNum, TotalFee, inbody, ref outputs); //第一代函数
int real = ZhiFuBaoPayCodeEx2(lockappid,registered, AuthCode, OrdNum, TotalFee, inbody, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",支付成功,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
case 1:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",正在支付中,请稍后查询支付状态,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",支付失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button6.Text = "支付宝付款码支付 Ex1";
}
private void button5_Click(object sender, EventArgs e)
{
string lockappid = ZFBAPPID.Text.Trim(); //支付宝APPID
int registered = 0; //registered = 0 原始的支付宝APPID =1 已加密授权的支付宝APPID
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string OrdNum = textBox2.Text.Trim();
string TotalFee =(Convert.ToDouble (textBox3.Text)/100).ToString ();
string inbody = textBox6.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
textBox1.Text = "";
textBox5.Text = "";
button5.Text = "正在申请支付URL...";
Application.DoEvents();
//int real = ZhiFuBaoPayQRCodeEx1(lockappid, OrdNum, TotalFee, inbody, ref outputs); //第一代函数
int real = ZhiFuBaoPayQRCodeEx2(lockappid,registered, OrdNum, TotalFee, inbody, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",支付二维码已经生成,有效时间5分钟,可轮询调用‘查询支付宝订单状态’函数查询支付结果!";
textBox5.Text = Marshal.PtrToStringAnsi(outputs).ToString();
pictureBox2.ImageLocation = Environment.CurrentDirectory + "\\QRCode.bmp";
break;
default:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",申请支付URL失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button5.Text = "生成支付宝支付二维码 Ex";
}
private void button11_Click(object sender, EventArgs e)
{
string lockappid = WXAPPID.Text.Trim(); //微信公众号
string lockmachid = WXMCHID.Text.Trim(); //商户号
int registered = 0; //registered = 0 原始的公众号、商户号 =1 已加密授权的公众号、商户号
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string idkey = WXKEY.Text.Trim(); //API密钥
int lockkey = 0; //lockkey = 0 原始API密钥 =1 加密后的API密钥
if (radioButton1.Checked == true) { lockkey = 0; } else { lockkey = 1; }
string OrdNum = textBox2.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
textBox1.Text = "";
button11.Text = "正在关闭微信订单...";
Application.DoEvents();
//int real = WeiXinCloseOrderEx1(lockappid, lockmachid, idkey, lockkey, OrdNum, ref outputs); //第一代函数
int real = WeiXinQueryEx2(lockappid, lockmachid,registered, idkey, lockkey, OrdNum, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "微信支付单号:" + OrdNum + ",关闭成功,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default:
textBox1.Text = "微信支付单号:" + OrdNum + ",关闭失败,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button11.Text = "关闭未支付的微信订单 Ex1";
}
private void button10_Click(object sender, EventArgs e)
{
string lockappid = ZFBAPPID.Text.Trim(); //支付宝APPID
int registered = 0; //registered = 0 原始的支付宝APPID =1 已加密授权的支付宝APPID
if (radioButton4.Checked == true) { registered = 0; } else { registered = 1; }
string OrdNum = textBox2.Text.Trim();
IntPtr outputs = Marshal.AllocHGlobal(255);
textBox1.Text = "";
button10.Text = "正在撒销支付宝订单...";
Application.DoEvents();
//int real = ZhiFuBaoPayCancelEx1(lockappid, OrdNum, ref outputs); //第一代函数
int real = ZhiFuBaoPayCancelEx2(lockappid,registered, OrdNum, ref outputs); //第二代函数
switch (real)
{
case 0:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",还未支付,已撒销成功!API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
case 1:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",撒销成功!已触发退款动作,API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
default:
textBox1.Text = "支付宝支付单号:" + OrdNum + ",撒销失败!API接口返回的信息是:" + Marshal.PtrToStringAnsi(outputs).ToString();
break;
}
button10.Text = "撒销支付宝订单 Ex1";
}
private void button9_Click_1(object sender, EventArgs e)
{
panel1.Visible = false;
}
}
}
C#微信、支付宝扫码支付源码
精选 原创
©著作权归作者所有:来自51CTO博客作者津津有味0202的原创作品,请联系作者获取转载授权,否则将追究法律责任
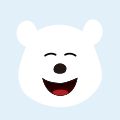
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Delphi7微信、支付宝扫码支付源码
Delphi7微信、支付宝扫码支付源码
Delphi7微信支付 Delphi7支付宝 Delphi7扫码支付 Delphi7二维码 Delphi7第三方支付 -
VFP开发微信、支付宝扫码支付
&& Vfp 微信、支付宝生
支付宝 微信 api接口 -
VB.net开发微信、支付宝扫码支付源码
扫码消费机介绍:https://item.taobao.com/item.htm?sp
Vb.net微信支付 Vb.net支付宝 Vb.net扫码支付 -
一条语句完成微信、支付宝扫码支付
微信、支付宝扫码支付动态库PayApdiFun.dll函数调用说明
微信支付 支付宝支付 扫码支付源码 PayApiFun