How to implement Drag and Drop from managed WinForm to Explorer
精选 转载The following code will enable dragging ListView items and dropping in Explorer to generate files. Temporary files will be generated and deferred rendering will be used...
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.IO;
namespace WinFormDragNDropExplorer
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.ListView listView1;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
System.Windows.Forms.ListViewItem listViewItem1 = new System.Windows.Forms.ListViewItem("Item 1");
System.Windows.Forms.ListViewItem listViewItem2 = new System.Windows.Forms.ListViewItem("Item 2");
System.Windows.Forms.ListViewItem listViewItem3 = new System.Windows.Forms.ListViewItem("Item 3");
this.listView1 = new System.Windows.Forms.ListView();
this.SuspendLayout();
//
// listView1
//
this.listView1.Items.AddRange(new System.Windows.Forms.ListViewItem[] {
listViewItem1,
listViewItem2,
listViewItem3});
this.listView1.Location = new System.Drawing.Point(40, 24);
this.listView1.Name = "listView1";
this.listView1.Size = new System.Drawing.Size(384, 280);
this.listView1.TabIndex = 0;
this.listView1.QueryContinueDrag += new System.Windows.Forms.QueryContinueDragEventHandler(this.listView1_QueryContinueDrag);
this.listView1.ItemDrag += new System.Windows.Forms.ItemDragEventHandler(this.listView1_ItemDrag);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(472, 333);
this.Controls.Add(this.listView1);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private ShellDataObject dataObj = null;
private void listView1_QueryContinueDrag(object sender, System.Windows.Forms.QueryContinueDragEventArgs e)
{
//ESC pressed
if (e.EscapePressed)
{
e.Action = DragAction.Cancel;
return;
}
//Drop!
if (e.KeyState == 0)
{
dataObj.SetData(ShellClipboardFormats.CFSTR_INDRAGLOOP, 0);
e.Action = DragAction.Drop;
return;
}
e.Action = DragAction.Continue;
}
private void listView1_ItemDrag(object sender, System.Windows.Forms.ItemDragEventArgs e)
{
String[] fileNames;
//Create the data object used for the drag drop operation
dataObj = new ShellDataObject();
//Create an array of strings to hold the filename(s)
fileNames = new String[listView1.SelectedItems.Count];
//Create temporary files that the user can drag.
for (int i = 0; i < listView1.SelectedItems.Count; i++)
{
fileNames[i] = CreateTemporaryFileName(listView1.SelectedItems[i].Text + ".txt");
}
//Add the list of files to the data object
dataObj.SetData(DataFormats.FileDrop, fileNames);
//Set the preferred drop effect
dataObj.SetData(ShellClipboardFormats.CFSTR_PREFERREDDROPEFFECT, DragDropEffects.Move);
//Indicate that we are in a drag loop
dataObj.SetData(ShellClipboardFormats.CFSTR_INDRAGLOOP, 1);
//Initiate the drag operation
listView1.DoDragDrop(dataObj, DragDropEffects.Move | DragDropEffects.Copy);
//Free the data object
dataObj = null;
}
private String CreateTemporaryFileName(String name)
{
String path;
String fileName;
System.IO.StreamWriter file;
//Build a path for the temporary file
path = System.IO.Path.GetTempPath();
fileName = System.IO.Path.Combine(path, name);
//Create the temporary file to make sure it exists
file = new System.IO.StreamWriter(fileName);
file.Close();
return fileName;
}
}
//Constant declarations. Please refer to "shlobj.h"
public class ShellClipboardFormats
{
public const string CFSTR_SHELLIDLIST = "Shell IDList Array";
public const string CFSTR_SHELLIDLISTOFFSET = "Shell Object Offsets";
public const string CFSTR_NETRESOURCES = "Net Resource";
public const string CFSTR_FILEDESCRIPTORA = "FileGroupDescriptor";
public const string CFSTR_FILEDESCRIPTORW = "FileGroupDescriptorW";
public const string CFSTR_FILECONTENTS = "FileContents";
public const string CFSTR_FILENAMEA = "FileName";
public const string CFSTR_FILENAMEW = "FileNameW";
public const string CFSTR_PRINTERGROUP = "PrinterFreindlyName";
public const string CFSTR_FILENAMEMAPA = "FileNameMap";
public const string CFSTR_FILENAMEMAPW = "FileNameMapW";
public const string CFSTR_SHELLURL = "UniformResourceLocator";
public const string CFSTR_INETURLA = CFSTR_SHELLURL;
public const string CFSTR_INETURLW = "UniformResourceLocatorW";
public const string CFSTR_PREFERREDDROPEFFECT = "Preferred DropEffect";
public const string CFSTR_PERFORMEDDROPEFFECT = "Performed DropEffect";
public const string CFSTR_PASTESUCCEEDED = "Paste Succeeded";
public const string CFSTR_INDRAGLOOP = "InShellDragLoop";
public const string CFSTR_DRAGCONTEXT = "DragContext";
public const string CFSTR_MOUNTEDVOLUME = "MountedVolume";
public const string CFSTR_PERSISTEDDATAOBJECT = "PersistedDataObject";
public const string CFSTR_TARGETCLSID = "TargetCLSID";
public const string CFSTR_LOGICALPERFORMEDDROPEFFECT = "Logical Performed DropEffect";
public const string CFSTR_AUTOPLAY_SHELLIDLISTS = "Autoplay Enumerated IDList Array";
}
public class ShellDataObject: DataObject
{
//This flag is used to prevent multiple callings to "GetData" when dropping in Explorer.
private bool downloaded = false;
public override object GetData(String format)
{
Object obj = base.GetData(format);
if (System.Windows.Forms.DataFormats.FileDrop == format &&
!InDragLoop() && !downloaded)
{
int i;
String[] files = (String[])obj;
for (i = 0; i < files.Length; i++)
{
DownloadFile(files[i]);
}
downloaded = true;
}
return obj;
}
private void DownloadFile(String filename)
{
StreamWriter file;
// Create the temporary file and its data
file = new StreamWriter(filename);
file.WriteLine(filename);
file.Close();
}
private bool InDragLoop()
{
return (0 != (int)GetData(ShellClipboardFormats.CFSTR_INDRAGLOOP));
}
}
}
上一篇:什么是程序员的优秀品质?
下一篇:我的友情链接
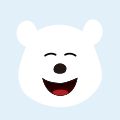
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
VARCHART XGantt甘特图如何Drag & Drop
VARCHART XGantt是一个交互式甘特图组件,可生成清晰,灵活的甘特图。本文介绍了甘特图Drag & Drop。
VARCHART XGantt 甘特图 XGantt -
Drag and Drop Grid (ExtJS - 2)
Sebagai lanjutan dari tutorial Extjs sebelumnya, sekarang saya mencoba untuk menuli
extjs html css javascript json