```import os
## os.system() #输出命令结果到屏幕,返回命令的执行状态
## os.popen("dir").read #会保存命令的执行结果并输出
# 在linux里面
import subprocess
subprocess.run(["ipconfig","ping 192.168.1.1"]) #同时执行多条命令
subprocess.run("df -h |grep book",shell=True) #把book文件过滤出来(涉及到管道符直接把字符串传给真正在调用的shell)
##subprocess模块常用命令
#执行命令,返回命令执行状态 , 0 or 非0 和os.sys一样
retcode = subprocess.call(["ls", "-l"])
#执行命令,如果命令结果为0,就正常返回,否则抛异常
subprocess.check_call(["ls", "-l"])
0
##(必须会用的)接收字符串格式命令,返回元组形式,第1个元素是执行状态,第2个是命令结果(有执行状态也有结果)
subprocess.getstatusoutput('ls /bin/ls')
(0, '/bin/ls')
#接收字符串格式命令,并返回结果
subprocess.getoutput('ls /bin/ls')
'/bin/ls'
#执行命令,并返回结果,注意是返回结果,不是打印,下例结果返回给res
res=subprocess.check_output(['ls','-l'])
res
b'total 0\ndrwxr-xr-x 12 alex staff 408 Nov 2 11:05 OldBoyCRM\n'
##把结果存在管道里,用stdout读取
res=subprocess.Popen("ifconfig|grep 192",shell=True,stdout=subprocess.PIPE)
res.stdout.read()
##stdout对屏幕的标准输出
##stdin 标准输入
##stderr 把错误单独分开
res=subprocess.Popen("ifcontttfig|grep 192",shell=True,stdout=subprocess.PIPE,stderr=subprocess.PIPE)
res.stdout.read() #因为命令错误所以读取为空
res.stderr.read() #读取错误信息
# poll()
res=subprocess.Popen("slppe 10;echo 'hello'",shell=True,stdout=subprocess.PIPE,stderr=subprocess.PIPE)
print(res.poll())#输出none代表命令程序没有执行完,返回0表示命令程序执行完。
res.wait() #等待命令执行完后返回0
res.terminate() #杀死正在执行程序,读取程序信息是空
# cwd
res=subprocess.Popen("slppe 10;echo 'hello'",shell=True,stdout=subprocess.PIPE,stderr=subprocess.PIPE,cwd="/temp")
res.stdout.read() #b'/tmp\n'新启动的shell目录所在的地方
#sudo自动输入密码
echo"123" | sudo -S apt-get install vim #自动输密码
subprocess.Popen("echo'123' | sudo -S apt-get install vim",shell=True) #在linux下调用python实现自动输入密码
subprocess模块
原创坚持和学习 ©著作权
文章标签 subprocess 模块 文章分类 Python 后端开发
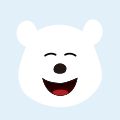
-
CentOS安装DataX
datax安装
datax