typedef int DataType; class SListNode { private: DataType _data; SListNode* next; friend class SList; }; class SList { private: SListNode* _head; SListNode* _tail; public: SList() :_head(NULL) , _tail(NULL) {} //SList(DataType x) // :_head(new SListNode) // , _tail(_head) //{ // _head->_data = x; // _head->next = NULL; //} //*******构造带参********简便******* SList(DataType x) { PushBack(x); } //********************** SList(SList& s) :_head(NULL) { SListNode* tmp = s._head; while (tmp) { PushBack(tmp->_data); tmp = tmp->next; } } //***********传统*************** //SList& operator=(SList& s) //{ // if (_head != s._head) // { // Clear(); // SListNode* cur = s._head; // while (cur) // { // PushBack(cur->_data); // cur = cur->next; // } // } // return *this; //} //***********现代*************** SList& operator=(SList s) { if (_head != s._head) { swap(_head, s._head); swap(_tail, s._tail); } return *this; } ~SList() { Clear(); } public: void PushBack(DataType x) { SListNode* tmp = new SListNode; tmp->_data = x; tmp->next = NULL; if (_head == NULL) { _head = tmp; _tail = _head; } else { _tail->next = tmp; _tail = _tail->next; } } void PopBack() { if (_head != NULL) { SListNode* tmp = _head; while (tmp->next != _tail) { tmp = tmp->next; } delete _tail; _tail = tmp; _tail->next = NULL; } } void Clear() { SListNode* tmp = _head; SListNode* del = _head; while (tmp) { del = tmp; tmp = tmp->next; delete del; del = NULL; } _head = NULL; } void PrintSList() { SListNode*tmp = _head; while (tmp) { cout << tmp->_data << "->"; tmp = tmp->next; } cout << "NULL" << endl; } };
单链表类成员函数现代写法、传统写法
原创
©著作权归作者所有:来自51CTO博客作者脚印C的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:双链表类中的逆置成员函数
下一篇:一个类如何只创建一个对象?
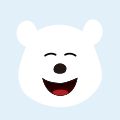
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
c++回调函数的几种写法(注意成员函数)
主要有3种写法。1.普通函数(全局函数)作为回调函数这
c++ 开发语言 c语言 回调函数 成员函数 -
C++ const成员变量和成员函数(常成员函数)
这种措施主要还是为了保护数据而设置的。co
c++ 开发语言 后端 成员变量 常成员函数